Introduction
In my previous post, explained about Share and Unshare Access which will trigger GrantAccess and also RevokeAccess. But those message, as explained before will only be triggered if you Share the record to User or Team that has no previous added access at all or also Unshare the record completely removing the access from the previous User or Team.How about if you want to have your own logic when you change the Access only to the specific users that already have the access, either you remove (not completely) and also add from the existing access so long you Update or Modify it, for End users, they just know about Share, but CRM has its own message request triggered.
The Code
And here is the code..*You can continue from my previous post code
else if (context.MessageName == "ModifyAccess") { // Obtain the target entity from the input parameter. EntityReference EntityRef = (EntityReference)context.InputParameters["Target"]; //after get this EntityRef then will be easy to continue the logic //Obtain the principal access object from the input parameter Microsoft.Crm.Sdk.Messages.PrincipalAccess PrincipalAccess = (Microsoft.Crm.Sdk.Messages.PrincipalAccess)context.InputParameters["PrincipalAccess"]; //Then got the User or Team and also Access Control that being Granted //***to Get User/Team that being Shared With var userOrTeam = PrincipalAccess.Principal; var userOrTeamId = userOrTeam.Id; var userOrTeamName = userOrTeam.Name; //this entityReference will not return name, only ID var userOrTeamLogicalName = userOrTeam.LogicalName; //use the logical Name to know whether this is User or Team! if (userOrTeamLogicalName == "team") { //what you are going to do if shared to Team? } if (userOrTeamLogicalName == "systemuser") { //what you are going to do if shared to Team? } Trace(userOrTeamId.ToString()); Trace(userOrTeamLogicalName); //***to Get the Principal Access var AccessMask = PrincipalAccess.AccessMask; Trace(AccessMask.ToString()); throw new InvalidPluginExecutionException("ModifyAccess triggered"); //please remove later //your logic continue here after already have all of them }
And here is the complete code
void Post_Message_GrantAccess(IServiceProvider serviceProvider, IPluginExecutionContext context) { if (context.MessageName == "GrantAccess") { //this GrantAccess is for sharing //for Unshare, messagename = "RevokeAccess" and will do the same passing parameters // Obtain the target entity from the input parameter. EntityReference EntityRef = (EntityReference)context.InputParameters["Target"]; //after get this EntityRef then will be easy to continue the logic //Obtain the principal access object from the input parameter Microsoft.Crm.Sdk.Messages.PrincipalAccess PrincipalAccess = (Microsoft.Crm.Sdk.Messages.PrincipalAccess)context.InputParameters["PrincipalAccess"]; //Then got the User or Team and also Access Control that being Granted //***to Get User/Team that being Shared With var userOrTeam = PrincipalAccess.Principal; var userOrTeamId = userOrTeam.Id; var userOrTeamName = userOrTeam.Name; //entityReference only return ID, not Name so will be empty string here var userOrTeamLogicalName = userOrTeam.LogicalName; //use the logical Name to know whether this is User or Team! if (userOrTeamLogicalName == "team") { //what you are going to do if shared to Team? } if (userOrTeamLogicalName == "systemuser") { //what you are going to do if shared to Team? } Trace(userOrTeamId.ToString()); Trace(userOrTeamLogicalName); //***to Get the Principal Access var AccessMask = PrincipalAccess.AccessMask; Trace(AccessMask.ToString()); //throw new InvalidPluginExecutionException("Shared"); //please remove later //your logic continue here after already have all of them } else if (context.MessageName == "RevokeAccess") { // Obtain the target entity from the input parameter. EntityReference EntityRef = (EntityReference)context.InputParameters["Target"]; //after get this EntityRef then will be easy to continue the logic //Unshare does not have PrincipalAccess because it removes all, only can get the revokee //Obtain the principal access object from the input parameter var Revokee = (EntityReference)context.InputParameters["Revokee"]; var RevokeeId = Revokee.Id; var RevokeeLogicalName = Revokee.LogicalName; //this one Team or User Trace(RevokeeId.ToString()); Trace(RevokeeLogicalName); throw new InvalidPluginExecutionException("Unshared"); //please remove later } else if (context.MessageName == "ModifyAccess") { // Obtain the target entity from the input parameter. EntityReference EntityRef = (EntityReference)context.InputParameters["Target"]; //after get this EntityRef then will be easy to continue the logic //Obtain the principal access object from the input parameter Microsoft.Crm.Sdk.Messages.PrincipalAccess PrincipalAccess = (Microsoft.Crm.Sdk.Messages.PrincipalAccess)context.InputParameters["PrincipalAccess"]; //Then got the User or Team and also Access Control that being Granted //***to Get User/Team that being Shared With var userOrTeam = PrincipalAccess.Principal; var userOrTeamId = userOrTeam.Id; var userOrTeamName = userOrTeam.Name; var userOrTeamLogicalName = userOrTeam.LogicalName; //use the logical Name to know whether this is User or Team! if (userOrTeamLogicalName == "team") { //what you are going to do if shared to Team? } if (userOrTeamLogicalName == "systemuser") { //what you are going to do if shared to Team? } Trace(userOrTeamId.ToString()); Trace(userOrTeamLogicalName); //***to Get the Principal Access var AccessMask = PrincipalAccess.AccessMask; Trace(AccessMask.ToString()); throw new InvalidPluginExecutionException("ModifyAccess triggered"); //please remove later //your logic continue here after already have all of them } }
Register the Plugin
And how to register the Plugin?Easy…
Just add more event so-called ModifyAccess
So for the complete solution, you will have 3 steps registered in the Plugin
1. GrantAccess
2. RevokeAccess
3. ModifyAccess
Result
And here is the result..I just want to modify the Access of this User from Read & Write to Read & Append & Share
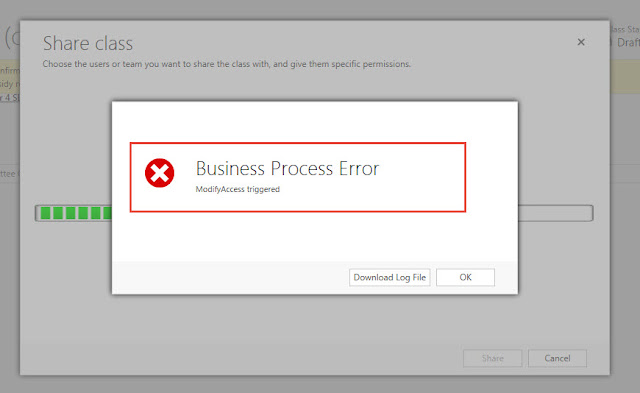
Then here is the Trace result
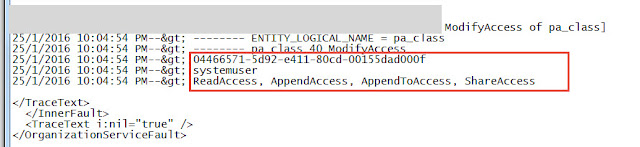
*Remember to always remove the “throw new InvalidPluginExecutionException” because it will stop your process.
And I hope this can help you!
This is the requirement in my project that currently I am doing it so basically is helping me to document it!